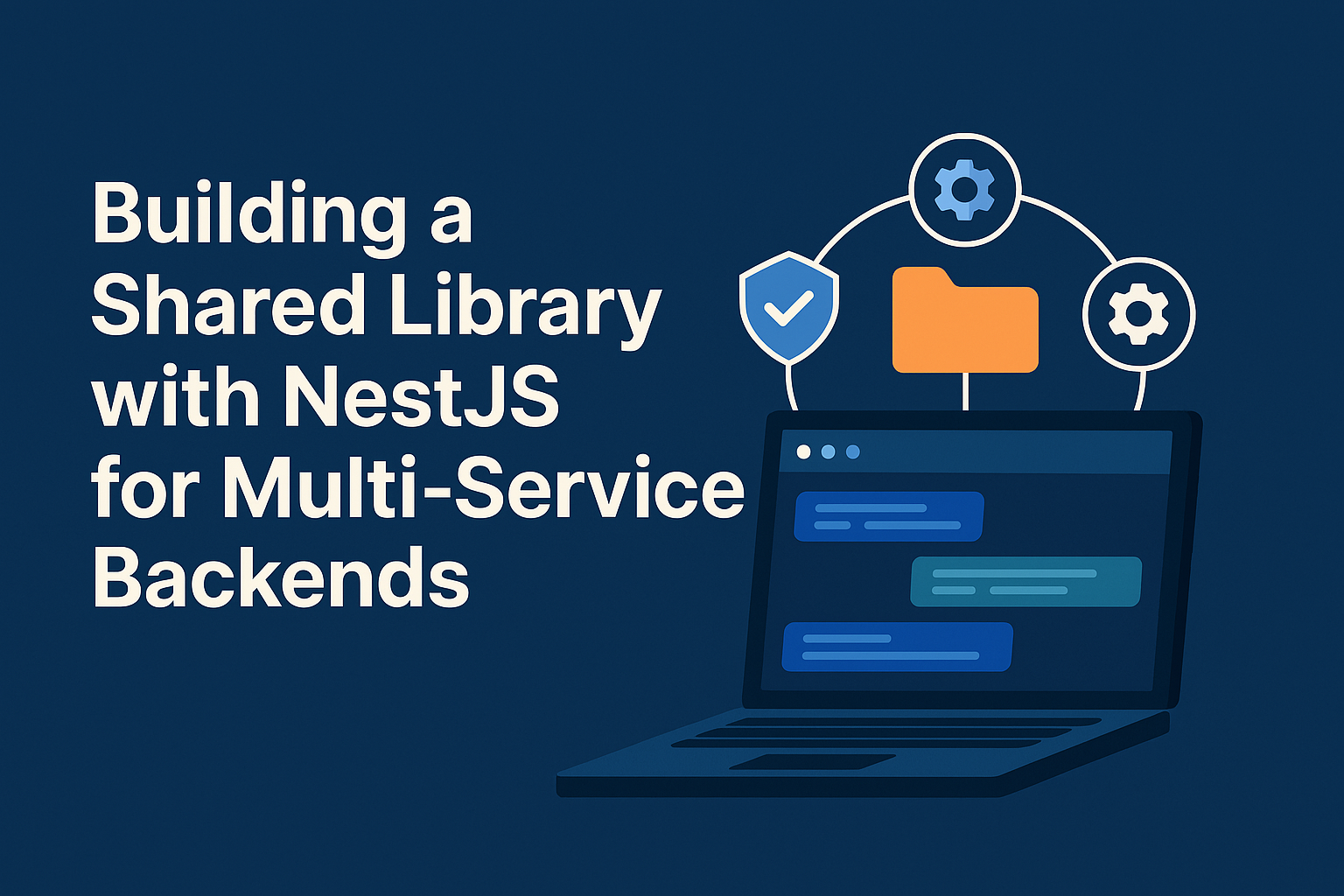
Building a Shared Library with NestJS for Multi-Service Backends
The no-fluff guide to reusing modules like Auth, Config, and Logging across your services.
So... you've got more than one NestJS service, huh?
Let me guess—you started with a single backend, maybe a monolith. Life was simple. Then came service boundaries, scaling concerns, and before you knew it, you had three, maybe four NestJS services, all eerily similar under the hood. Same auth logic. Same config setup. Same logging wrapper that you copy-pasted one too many times.
Feels like déjà vu with extra steps, right?
That’s where a shared library comes in—not just as a way to avoid duplication, but as a way to bring sanity, consistency, and a bit of joy back to your dev workflow.
Let’s break it down.
“Shared library” sounds fancy. What is it really?
It’s just code you reuse—centralized modules, services, interfaces, and utilities—bundled neatly, versioned properly, and imported wherever you need them. Think of it like your own internal toolkit, built to match your needs.
You might be sharing things like:
- An authentication module, including strategies, guards, and decorators.
- A configuration module that handles your environment parsing and secret management.
- A logging module tailored to your preferred formatting and logging levels.
- Shared data transfer objects, filters, interceptors, and maybe even utilities.
The real kicker? You stop fixing the same bug in five different places.
Monorepo vs Multi-repo: Does it matter?
Honestly? Kind of. But not in the way people argue about on Twitter.
If you’re in a monorepo—maybe using Nx or yarn workspaces—it’s super straightforward. You keep your shared library in a dedicated folder, and your other services can reference it directly. No packaging required.
But if you’re in a multi-repo setup? That’s where things get spicier. You’ll need to package and publish your shared code somewhere—maybe a private npm registry, or through Git submodules (not for the faint of heart). Either way, version control becomes way more important.
To sum it up: monorepos are easier. Multi-repos are totally possible, just a bit more work.
Building the thing: Let’s talk structure
Here’s a simple structure that works well without going overboard:
You’d have a top-level folder for shared libraries. Inside that, maybe one for authentication, one for configuration, and one for logging. Each one contains its relevant modules and helper files. At the root of each folder, have a single entry file that determines what’s publicly available.
If you’re using Nx, you can even generate these libraries with commands that set up boilerplate and testing for you.
A word about dependencies (because this one trips people up)
Let’s say your shared authentication module uses a third-party package like Passport. Instead of embedding that package directly as a dependency inside the shared library, make it a peer dependency. That way, each service using the shared module is responsible for installing the package itself. It avoids bugs caused by version mismatches or duplicate installations.
Also, try not to let your shared libraries manage stateful things like database connections. That’s a fast track to confusing behavior. Think of shared libraries as tools, not managers.
Publishing and Versioning: Simple is smart
If you're working in a monorepo, you can keep all libraries on the same version and bump them together. Tools like Changesets help automate this, especially when used in CI.
In multi-repo setups, versioning happens per package. When you update something, you’ll want to decide if it’s a patch, a minor update, or a major release. Patch updates are for bug fixes, minor updates for backwards-compatible features, and major versions for breaking changes.
Publishing can be automated via CI/CD. But no matter what, keep a changelog. It may feel tedious at first, but it pays off big when debugging or onboarding someone new.
One last thing—don’t sneak breaking changes into small updates. That’s how trust dies.
What should you actually share?
Use this as a loose checklist:
- Are two or more services using this code?
- Is it relatively stable—not something that changes every sprint?
- Does it serve a foundational purpose like config, auth, or logging?
If yes, move it to the shared library.
But if it’s business logic that only applies to one service? Keep it local. Sharing for the sake of sharing leads to headaches.
A few things that might bite you
Let’s talk about some common pitfalls.
- Circular dependencies can sneak in if your shared modules import each other. Keep them isolated and flat.
- Environment-specific behavior may lead to unexpected results. Test your shared modules in every service that uses them.
- Version drift can happen in multi-repo setups. One service uses version one-point-two, another clings to version one-point-zero, and suddenly your shared code behaves inconsistently.
- Over-abstraction is tempting but dangerous. If your shared code tries to account for every possible use case, you’ll end up rewriting it constantly.
Messing up is fine—just learn and move forward.
Final thoughts (no big moral, just vibes)
This whole shared-library thing? It’s not about writing fancy code for its own sake. It’s about giving yourself and your team less to worry about.
Less repetition. Fewer bugfixes. More time for things that matter.
So if you’re still copy-pasting the same modules across your NestJS services, maybe it’s time.
Start small. Make things boring. Let the library do the work.
And if all goes well, you’ll forget the shared code even exists. That’s how you know it’s working.