April 3, 2025
Test Driven Development with NestJS: Write Less, Think More
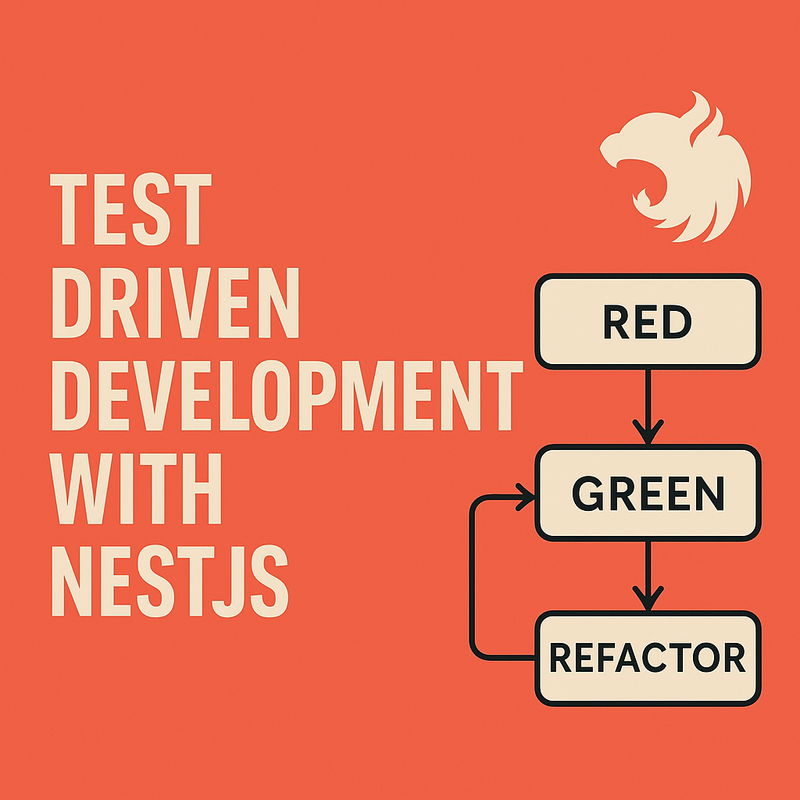
April 3, 2025
Test driven development—or TDD if you're tired of typing it out—sounds like a philosophy, but it's really just a habit. You write a test. It fails. You write code to make it pass. Then maybe you clean it up a bit.
That’s it. No magic. No ceremonies.
But if you do it consistently, it changes how you think about building software. And in frameworks like NestJS, it actually feels... doable.
So let’s talk about what makes TDD worth your time (and sometimes your frustration), how it fits into a NestJS backend, and why it helps keep your code sane when everything else is moving fast.
Honestly? Because otherwise you probably won’t write it at all. Or you’ll write it later, after the code’s grown weird edges and dependencies, and now you’re just testing that it doesn’t crash—rather than what it’s supposed to do.
Writing the test first means you have to define what success looks like before you write any logic. It forces clarity.
It’s like writing the punchline before the joke. You already know the payoff. Now you just have to build the setup that makes it land.
That sounds strange, but it makes your code tighter. You stop writing "just in case" logic. You’re focused. You're writing code for a reason.
NestJS can be a little opinionated, sure—but that’s kind of the point. It pushes you toward separation of concerns, modules, dependency injection... all the stuff that makes testing easier (whether you’re into it or not).
Plus, it’s built with TypeScript. And TypeScript, with its strong typing and predictable interfaces, pairs naturally with TDD. You get faster feedback and fewer surprises.
And NestJS comes bundled with Jest—so no fiddling with setup. You write a test, run it, and it just works. For once, the defaults are actually helpful.
You’ve probably seen the loop before:
Red → Green → Refactor.
That’s the whole thing. Write a failing test (red). Write code that passes the test (green). Then tidy things up (refactor).
But in real life, it plays out more like this:
Then you do it again.
The key is staying small. Don’t get clever. Don’t try to predict the next five steps. Just solve one thing. Then move on.
Here’s the part folks don’t like to say out loud—TDD does slow you down in the beginning. That’s normal. You’re pausing more. You’re thinking harder before writing code. You’re building discipline.
But after a while, something changes.
You’re not spending hours debugging weird side effects. You’re not afraid to refactor something risky. You’re not writing throwaway logic that gets deleted next week. Your code starts behaving itself.
It’s like working out. At first, everything hurts. Then you start moving better.
Picture this. You’re building a UserService. It needs a method to fetch a user by ID.
So you write a test that says, “When I call this method with an ID, it returns a user object.”
The test fails, because the method doesn’t exist yet. Good.
Now you add the method. Maybe it uses Prisma. Maybe you mock the database so your test doesn’t care about real data.
You get it passing. You clean it up. Done.
Later, someone wants to change how users are stored. Great. Your test doesn’t care. You can refactor the storage logic and still be sure the method returns the right result.
That’s the payoff: flexibility without fear.
One mistake people make with TDD is overdoing it. Not everything needs a test. You don’t need a test for a function that returns true if a number is greater than 5. You could write one—but should you?
Focus on behavior that matters.
Write tests that answer those questions.
Skip the ones that exist just to make your coverage tool happy.
Here’s something nobody told me early enough: you’re allowed to fake stuff.
If your service talks to a database, don’t hit the real thing. Mock it. Just pretend.
If your controller returns a value from a service, don’t build the full service for the test. Stub it. Provide the expected output.
NestJS makes this easy with dependency injection. You can swap out real providers with fake ones during testing. No need to spin up Docker or seed a database just to check if a string equals another string.
Keep your tests fast, clean, and focused. Realistic? Sure. But not too real.
Sometimes you’re building something fuzzy. A UI component. A vague product concept. Something you’re still figuring out. In those cases, TDD can feel like walking through mud.
That’s fine.
You can explore first, then write tests once things settle. TDD isn’t a religion. It’s a tool.
Use it when it helps. Don’t use it when it doesn’t.
TDD isn’t magic. It doesn’t write code for you. It doesn’t protect you from deadlines or messy specs or awkward meetings.
But it does this one thing really well: it forces you to slow down and think before you act. And in backend code—especially the kind of code that lives for years and gets passed from team to team—that’s huge.
With NestJS, the whole setup nudges you in the right direction. You’ve got modules. You’ve got services. You’ve got test scaffolding baked in.
So maybe give it a shot. Start with one small thing. Write the test first. Make it pass. Clean it up. Repeat.
You might find yourself writing less code—but better code. And you’ll definitely sleep a little easier knowing your app isn’t held together by duct tape and good intentions.
And hey, if nothing else, writing tests first means fewer bugs during your next on-call shift. And that’s worth plenty.